Overview
The getProperty, and setProperty APIs allow you to gather data stored in your project and alter it as you please through JavaScript code. This can be useful if you would like a project to change in appearance with a simple call to an Action, or if you want to gather information regarding what your user is typing or viewing. In this example, we will register an Action that will change the page information from that of a vanilla frosted donut to that of a chocolate frosted donut and vice versa by getting and setting properties for a Text Component, a Text Entry Component, and an Image Component.
Prerequisites
- Umajin Editor downloaded and installed.
- Any text editor.
- Completed the registerAction tutorial.
Instructions
Step 1) Create a new blank project in the Umajin Editor to use as a testing platform for our property altering platform.
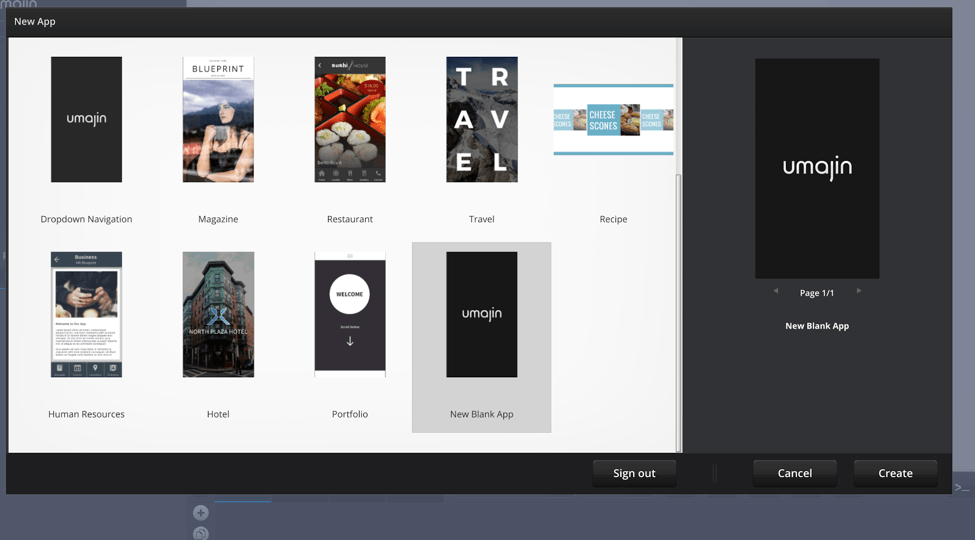
Step 2) Create a new JavaScript document and name it something that relates to your desired action. For our example, we will be naming the file GetSetProperty.js because our goal is to demonstrate the use of getting and setting the properties of three different components: Text, Text Entry, and Image. Make sure that you save this file in the scripts folder under the Umajin Resources Folder.
File → Open Resources Folder → scripts
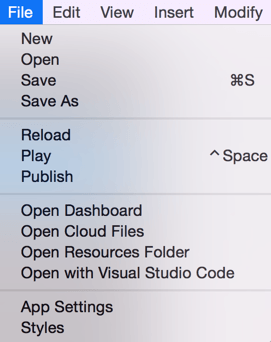
Step 3) We can now set up our Umajin page for our testing example. On our page, we have a Text Component serving as a header, a Text Entry Component that will prompt the user to describe the donut, and an Image Component displaying a donut. Our base page uses information regarding a vanilla frosted donut. There is also a Button Component which our Action will be tied to later on. As you can see, the default text is “Vanilla Donut”, the default text empty prompt is “PLEASE DESCRIBE THE VANILLA DONUT HERE”, and the default image is “VanillaDonut.jpg”. You are welcome to use both donut image files attached below.
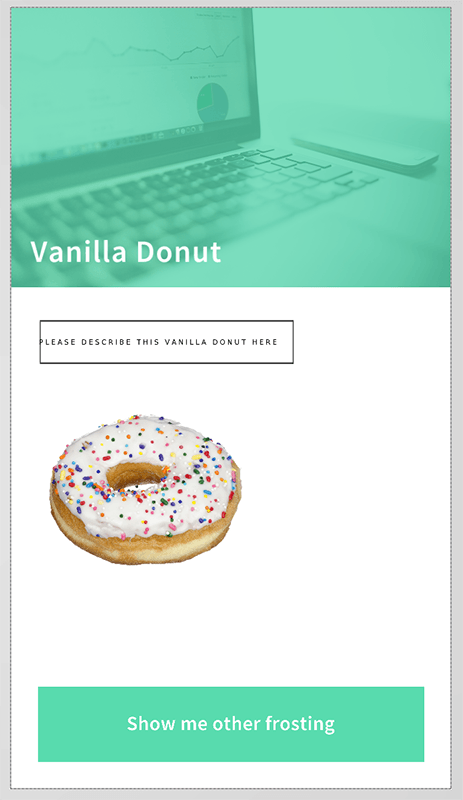
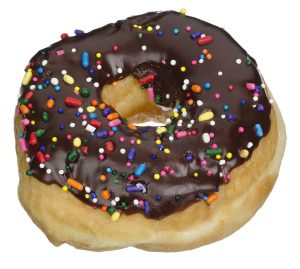
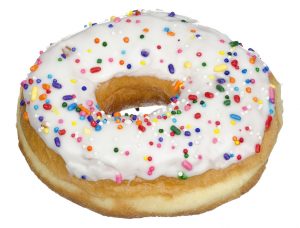
Step 4) Now we can open up our JavaScript file, and register our Action. This step is described in greater detail in our registerAction tutorial. We will call our method “changeFrosting” and it will have three Components: our Text, Text Entry, and Image.
registerAction("changeFrosting",'', "Donut Frosting Action","component:text:text,component:text_entry:text_entry,component:image:image")
Step 5) We can now write our changeFrosting method. To make it easier to follow, we will break this into a few sub-steps. This step will introduce both our getProperty and setProperty APIs.
getProperty takes in two parameters. The first is the object that you will be retrieving properties from. The second is what property you hope to retrieve, which will be taken in as a string. At the very bottom of this tutorial, you can find a list of properties that can be retrieved from certain object components.
setProperty takes in three parameters, the first two are the same as getProperty and the third is what you would like to set that property to. This will almost always be a string type.
- Define the function with parameters corresponding to those listed in our registerAction line. We will save the donut type label, which will be in the text component, as a local variable.
function changeFrosting(text, text_entry, image){ var textboxText = getProperty(text, "text");
- Write the if statement that will switch the heading of the page. If the heading represents a vanilla donut, we will change it to chocolate, and vice versa.
if(textboxText == "Vanilla Donut"){ setProperty(text,"text","Chocolate Donut"); }else{ setProperty(text,"text","Vanilla Donut"); } }
- Add to the if statement two lines of code that will switch the text entry prompt on the page. If the prompt request’s information for a vanilla donut, we change it to a request for chocolate donut information and vice versa.
if(textboxText == "Vanilla Donut"){ setProperty(text,"text","Chocolate Donut"); setProperty(text_entry,"text","PLEASE DESCRIBE THIS CHOCOLATE DONUT HERE"); }else{ setProperty(text,"text","Vanilla Donut"); setProperty(text_entry,"text","PLEASE DESCRIBE THIS VANILLA DONUT HERE"); } }
- Add to the if statement two lines of code that will switch the image on the page. If the image is of a Vanilla Donut, switch it to that of a chocolate donut and vice versa.
if(textboxText == "Vanilla Donut"){ setProperty(text,"text","Chocolate Donut"); setProperty(text_entry,"text","PLEASE DESCRIBE THIS CHOCOLATE DONUT HERE"); setProperty(image,"filename","ChocolateDonut.jpg"); }else{ setProperty(text,"text","Vanilla Donut"); setProperty(text_entry,"text","PLEASE DESCRIBE THIS VANILLA DONUT HERE"); setProperty(image,"filename","VanillaDonut.jpg"); } }
Step 6) Make sure that your new Action is called with the use of your Button.
Click the button → Actions → On Press → Donut Frosting Action
Step 7) Test our your code by pressing the play button in Umajin. After we press out button, this is what the project looks like:
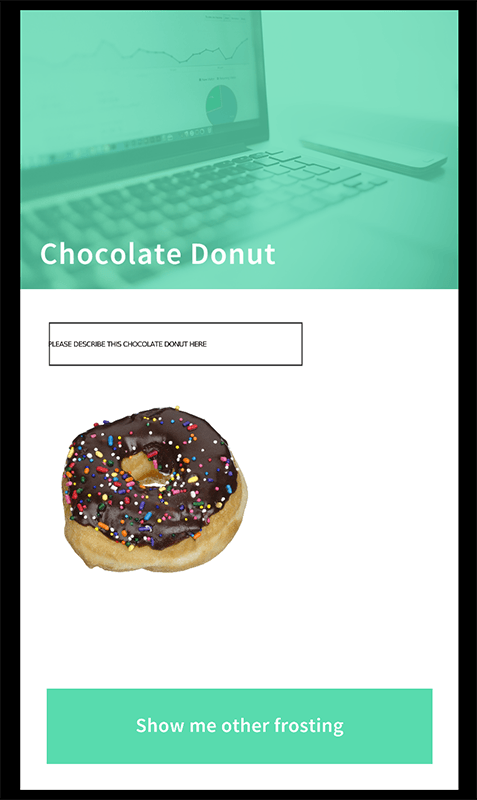
Properties
(All of type string)
Name | Description |
width | any component |
height | any component |
native_width | text, html_article, image |
native_height | text, html_article, image |
x | any component |
y | any component |
visible | any component |
lock_left | any component |
lock_right | any component |
lock_width | any component |
lock_height | any component |
lock_top | any component |
lock_bottom | any component |
instance_display_name | any component |
unique_id | any component |
text | text, text_entry, html_article |
font_color | text, button |
json | custom component |
scroll_offset | any feed list or scroll panel |
scroll_min | feed list |
scroll_max | feed list |
filename | image, video, animation |
instance_display_name | any component |